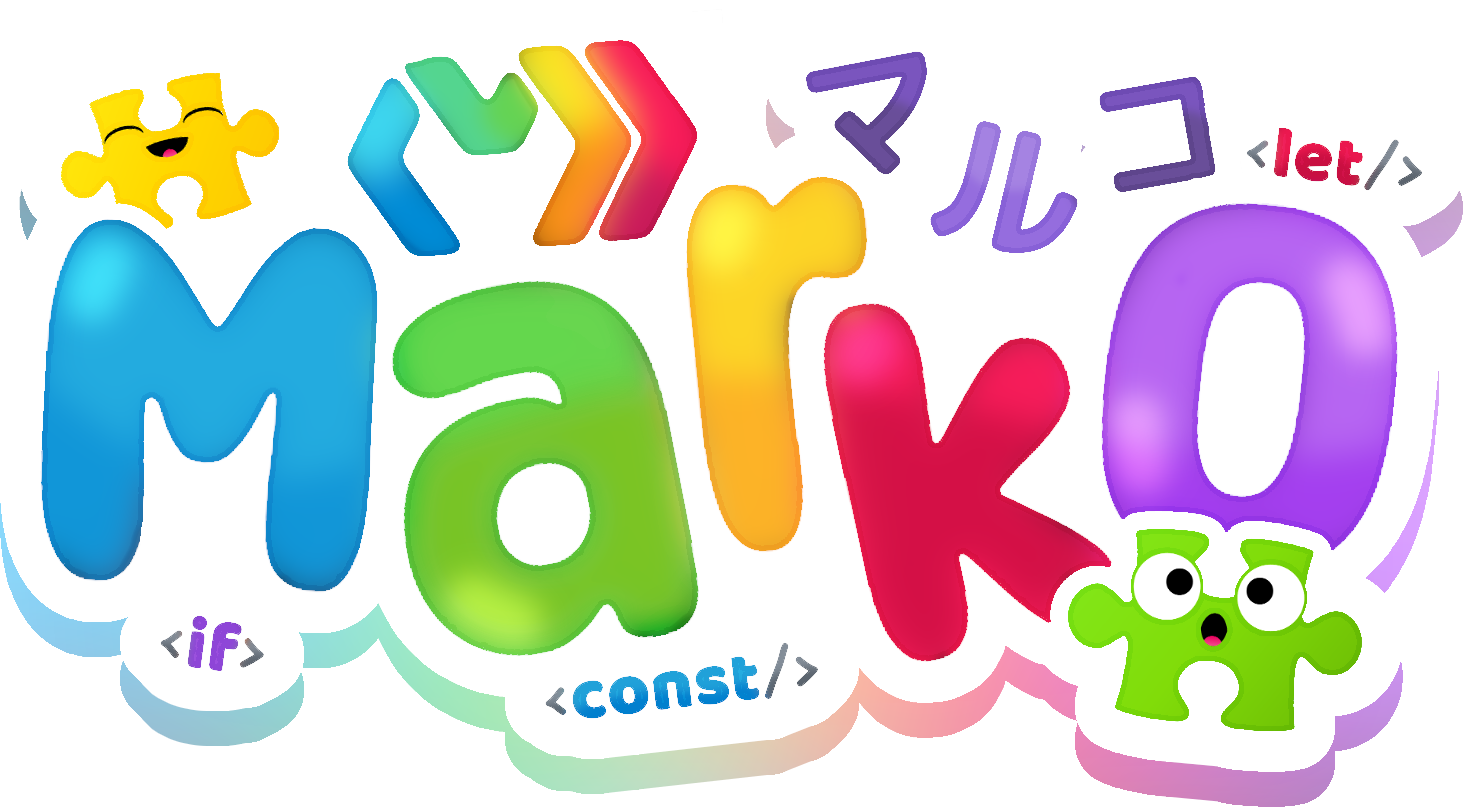
Marko + Express
Quick Start
npm init marko -- --template vite-express # Or `npx create-marko --template vite-express`
See Marko’s example projects for working Express integration code, such as:
But if you want to do things the hard way…
Doing things the hard way
First, install Marko, Express, and the glue to hold them together:
npm install marko express @marko/express --save
Skip the view engine
Express’s builtin view engine may be asynchronous, but it doesn’t support streaming — see Rediscovering Progressive HTML Rendering for why that’s important. So instead, we bypass Express’s view engine to use @marko/express
instead.
The @marko/express
package adds a res.marko()
method to Express’s response object. This method works like res.render()
, but without the restrictions of Express’s view engine, letting you take full advantage of Marko’s streaming and modular template organization.
ProTip: By using
res.marko()
, properties fromapp.locals
andres.locals
are automatically available on$global
.
import express from "express"; import markoPlugin from "@marko/express"; import template from "./template.marko"; const app = express(); app.use(markoPlugin()); // Enables `res.marko(template, input)` app.get("/", function (req, res) { res.marko(template, { name: "Frank", count: 30, colors: ["red", "green", "blue"], }); }); app.listen(8080);
Note: Older versions of
@marko/express
used to also attach Express’sapp
,req
, andres
objects onto$global
. This meant uncontrolled network data could cause new and exciting surprises in your app code. Nowadays we recommend explicitly accessing the specific pieces of the HTTP exchange you’re interested in, like this:app.get("/", function (req, res) { res.marko(template, { params: req.params, submitted: req.method === "POST" && req.body, }); });
BYOB (Bring Your Own Bundler)
Most of Marko’s API requires a bundler: the example code above assumes that .marko
files can be import
ed in your environment. Check out Marko’s supported bundlers to see what works best for you.
Contributors
Helpful? You can thank these awesome people! You can also edit this doc if you see any issues or want to improve it.